First of all, do File New, but this time do not select File New - Web Site, but select File New - Project instead. As one of the New Project targets, we can select Web and then pick an ASP.NET Web Application, as can be seen below.
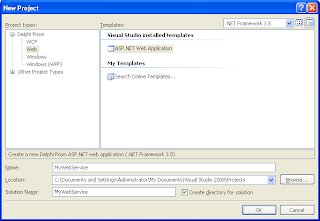
Note that we can select the (minimum) version of the .NET Framework for this project (3.5 in the screenshot above), and unlike the Web Site, we cannot select HTTP as location, but need to specify a normal location for the Web Project. When we deploy the project, we can select a HTTP location as we'll see later in this section. For the new example, select MyWebService as Name for the solution as well as the project. Note that this will produce an ASP.NET Web Application, and not directly an ASP.NET Web Service, but this can be "fixed" after the project is created.
After we click on OK, a new ASP.NET Web Application project called MyWebService is created (in a solution which is also called MyWebService). The Solution Explorer shows the project with a Default.aspx web page, as well as a Global.asax (for the ASP.NET application object) and a web.config file. We don't need the Default.aspx and related source files, so open the node in the Solution Explorer, select the three files Default.aspx, Default.aspx.designer.pas as well as Default.aspx.pas, right-click on the nodes and select Remove. In the dialog that follows, you can click on Delete to permanently delete Default.aspx (and the related .pas files).
Without the Default web page, the project is only ASP.NET and needs another main item. Right-click on the MyWebService project node, and select "Add - New Item. In the dialog that follows, we need to go to the Web category and select a Web Service.
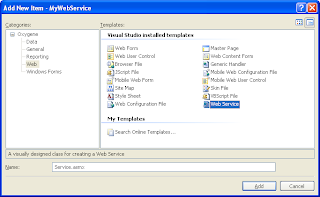
Change the name of the Web Service from WebService1.asmx to Service.asmx and click on Add.
First, modify the namespace attribute again,
type
[WebService(&Namespace := 'http://eBob42.org/',
Description := 'This web service is a new demo Web Service<br>' +
'generated by <b>Delphi Prism</b> for ASP.NET 2.0 or higher')]
[WebServiceBinding(ConformsTo := WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from script, using ASP.NET
// AJAX, uncomment the following line.
// [System.Web.Script.Services.ScriptService]
Service = public class(System.Web.Services.WebService)
public
Note the new comment about the System.Web.Script.Services.ScriptService, to allow the web service to be called from JavaScript, a topic for a future article.
EnableSession
Then, we should remove the demo method HelloWorld, and add four new WebMethods.
All of them with the EnableSession attribute set to true, to enable the use of the ASP.NET session object for these four web methods:
Service = public class(System.Web.Services.WebService)
public
[WebMethod(EnableSession := true)]
method Remember(const Name,Value: String);
[WebMethod(EnableSession := true)]
method Recall(const Name: String): String;
[WebMethod(EnableSession := true)]
method Forget(const Name: String);
[WebMethod(EnableSession := true)]
method Amnesia; // forget all
end;
Press Ctrl+Shift+C to generate the skeletons for the four web methods, and implement them as follows:
method Service.Remember(const Name,Value: String);
begin
Session[Name] := Value
end;
method Service.Recall(const Name: String): String;
begin
if Assigned(Session[Name]) then
Result := Session[Name].ToString
else Result := ''
end;
method Service.Forget(const Name: String);
begin
Session.Remove(Name)
end;
method Service.Amnesia;
begin
Session.Abandon
end;
The implementation of the four web methods is relative straightforward, using the ASP.NET Session object to store the name-value combinations, or clear them (or clear everything, by calling the Session.Abandon method).
We can now run the server in a browser using Debug Start without Debugging.
This will start the ASP.NET Deployment Server (at http://localhost:2743 on my machine), showing the web service on our localhost.
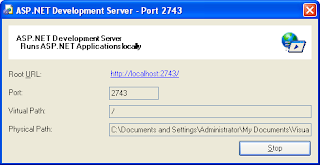
If you didn't selected Service.asmx as Start Page (by right-clicking on it in the Solution Explorer), you may need to manually add Service.asmx to the URL in the browser. Then, you can use the browser to test the web service. For example, you can click and execute the Remember method and make the web service remember a name-value combination, which you can then use to test Recall (or Forget). This should all work just fine, preparing the way for the actual web service client.
Client
Although you may want to deploy the web service before releasing the client, we can already create a client application and allow it to consume the web service part of the same solution.
First, let's create a new Windows Application, call it WindowsClientApplication.
No comments:
Post a Comment